Insane Limits V0.9/R4: Clan Tag Leaderboard (2 teams, not Rush, not CTF)
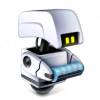
By
ImportBot
in Plugin Enhancements
-
Our picks
-
EZRCON Update (9/4/2021)
Prophet731 posted a topic in News,
Game Server Hosting:
We're happy to announce that EZRCON will branch out into the game server provider scene. This is a big step for us so please having patience if something doesn't go right in this area. Now, what makes us different compared to other providers? Well, we're going with the idea of having a scaleable server hosting and providing more control in how you set up your server. For example, in Minecraft, you have the ability to control how many CPU cores you wish your server to have access to, how much RAM you want to use, how much disk space you want to use. This type of control can't be offered in a single service package so you're able to configure a custom package the way you want it.
You can see all the available games here. Currently, we have the following games available.
Valheim (From $1.50 USD)
Rust (From $3.20 USD)
Minecraft (Basic) (From $4.00 USD)
Call of Duty 4X (From $7.00 USD)
OpenTTD (From $4.00 USD)
Squad (From $9.00 USD)
Insurgency: Sandstorm (From $6.40 USD)
Changes to US-East:
Starting in January 2022, we will be moving to a different provider that has better support, better infrastructure, and better connectivity. We've noticed that the connection/routes to this location are not ideal and it's been hard getting support to correct this. Our contract for our two servers ends in March/April respectively. If you currently have servers in this location you will be migrated over to the new provider. We'll have more details when the time comes closer to January. The new location for this change will be based out of Atlanta, GA. If you have any questions/concerns please open a ticket and we'll do our best to answer them.-
- 5 replies
Picked By
Prophet731, -
-
EZRCON Update 5/13/2021
Prophet731 posted a topic in News,
Hello All,
I wanted to give an update to how EZRCON is doing. As of today we have 56 active customers using the services offered. I'm glad its doing so well and it hasn't been 1 year yet. To those that have services with EZRCON, I hope the service is doing well and if not please let us know so that we can improve it where possible. We've done quite a few changes behind the scenes to improve the performance hopefully.
We'll be launching a new location for hosting procon layers in either Los Angeles, USA or Chicago, IL. Still being decided on where the placement should be but these two locations are not set in stone yet. We would like to get feedback on where we should have a new location for hosting the Procon Layers, which you can do by replying to this topic. A poll will be created where people can vote on which location they would like to see.
We're also looking for some suggestions on what else you would like to see for hosting provider options. So please let us know your thoughts on this matter.-
- 4 replies
Picked By
Prophet731, -
-
Procon Version 1.5.3.5 Released
Prophet731 posted a topic in News,
Added ability to disable the new API check for player country info
Updated GeoIP database file
Removed usage sending stats
Added EZRCON ad banner
If you are upgrading then you may need to add these two lines to your existing installation in the file procon.cfg. To enable these options just change False to True.
procon.private.options.UseGeoIpFileOnly False
procon.private.options.BlockRssFeedNews False
-
- 2 replies
Picked By
Prophet731, -
-
Procon, Database & BFACP Hosting
Prophet731 posted a topic in News,
I wanted I let you know that I am starting to build out the foundation for the hosting services that I talked about here. The pricing model I was originally going for wasn't going to be suitable for how I want to build it. So instead I decided to offer each service as it's own product instead of a package deal. In the future, hopefully, I will be able to do this and offer discounts to those that choose it.
Here is how the pricing is laid out for each service as well as information about each. This is as of 7/12/2020.
Single MySQL database (up to 30 GB) is $10 USD per month.
If you go over the 30 GB usage for the database then each additional gigabyte is charged at $0.10 USD each billing cycle. If you're under 30GB you don't need to worry about this.
Databases are replicated across 3 zones (regions) for redundancy. One (1) on the east coast of the USA, One (1) in Frankfurt, and One (1) in Singapore. Depending on the demand, this would grow to more regions.
Databases will also be backed up daily and retained for 7 days.
Procon Layer will be $2 USD per month.
Each layer will only allow one (1) game server connection. The reason behind this is for performance.
Each layer will also come with all available plugins installed by default. This is to help facilitate faster deployments and get you up and running quickly.
Each layer will automatically restart if Procon crashes.
Each layer will also automatically restart daily at midnight to make sure it stays in tip-top shape.
Custom plugins can be installed by submitting a support ticket.
Battlefield Admin Control Panel (BFACP) will be $5 USD per month
As I am still working on building version 3 of the software, I will be installing the last version I did. Once I complete version 3 it will automatically be upgraded for you.
All these services will be managed by me so you don't have to worry about the technical side of things to get up and going.
If you would like to see how much it would cost for the services, I made a calculator that you can use. It can be found here https://ezrcon.com/calculator.html
-
- 11 replies
Picked By
Prophet731, -
-
Procon Version 1.5.3.4 Released
Prophet731 posted a topic in News,
I have pushed out a new minor release which updates the geodata pull (flags in the playerlisting). This should be way more accurate now. As always, please let me know if any problems show up.
-
- 9 replies
Picked By
Prophet731, -
-
Recommended Posts
Archived
This topic is now archived and is closed to further replies.